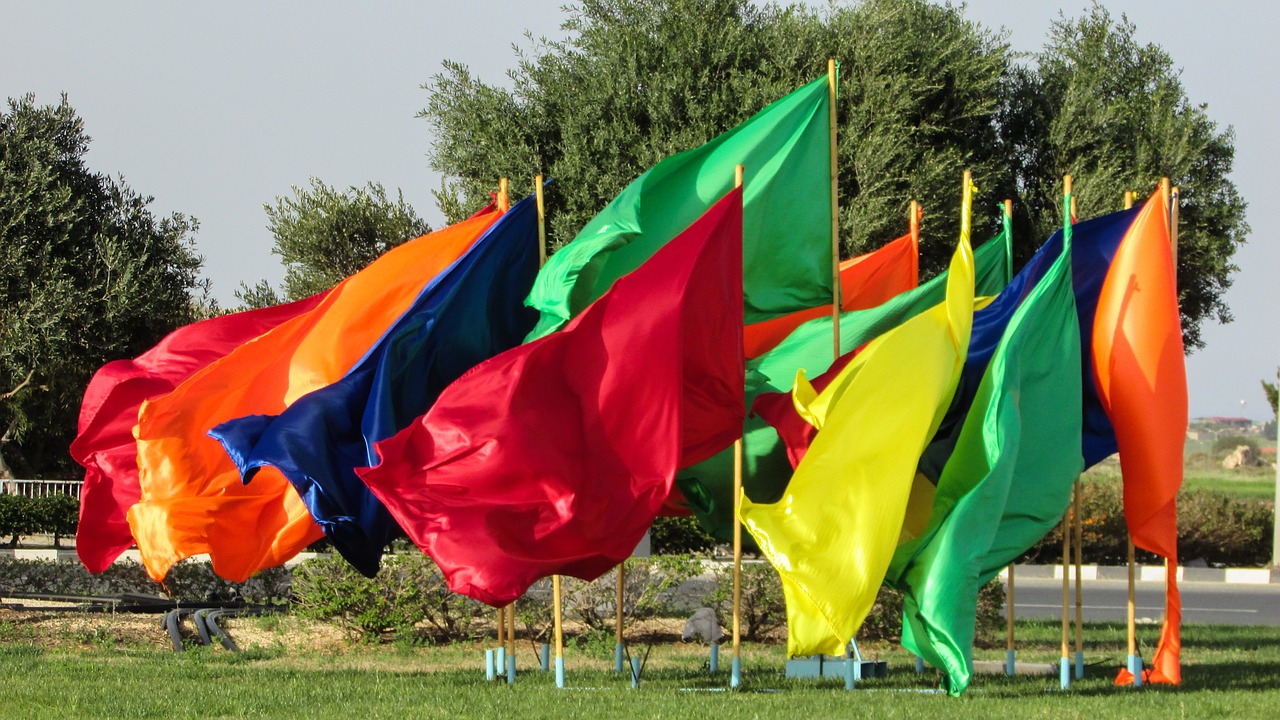
Enum flags in C# are life
For the 5 years I’ve been working on and off with C# I’ve never encountered enum flags. Until yesterday. I thought the concept would make a great blog post so here it is!
Yesterday’s approach to enums
If you asked me to hold multiple enums in a variable here is how I would’ve done it before my enlightenment:
using System;
using System.Collections.Generic;
public class HelloWorld
{
static public void Main ()
{
Console.WriteLine("Hello Mono World");
List<Fruits> basket = new List<Fruits>()
{
Fruits.Apples,
Fruits.Watermelons,
Fruits.Pears
};
Console.Write("In our basket we have: ");
foreach (Fruits fruits in basket)
{
Console.Write(fruits + " ");
}
}
}
public enum Fruits
{
Apples,
Bannanas,
Watermelons,
Raspberries,
Pears
}
The new ways
Since we are learning every day here is how we could perform something similar with enum flags:
using System;
public class HelloWorld
{
static public void Main ()
{
Console.WriteLine("Hello Mono World");
Fruits basket = Fruits.Apples | Fruits.Watermelons;
Console.WriteLine($"In our basket we have {basket}");
}
}
[Flags]
public enum Fruits
{
Apples = 1,
Bannanas = 2,
Watermelons = 4,
Raspberries = 8,
Pears = 16
}
The nice thing about flags is that C# will automatically print out the name of all the enums instead of the number. Note that the values of enums are all powers of 2, ensuring that the number stored in a variable (in our case basket) is unique.
I hope this post was quite informative for you. Please remember to ABC (Always Be Coding)!